Mastering Face Recognition with OpenCV and Python
Written on
Chapter 1: Introduction to Face Recognition
In a previous post, I discussed the procedure for installing OpenCV on your Mac system. Today, we’re diving into a practical application of OpenCV: recognizing faces in images.
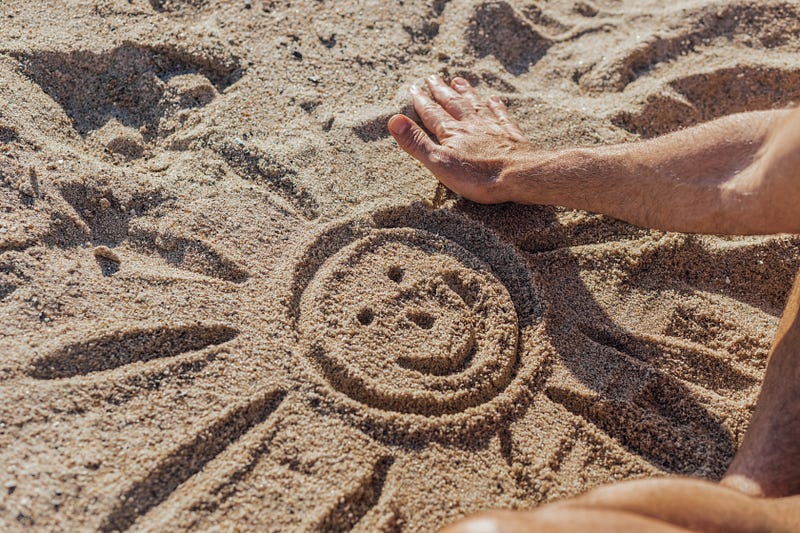
Setting Up OpenCV for Python on macOS
This method is compatible with both M1 and Intel architectures.
To get started, ensure you have Miniforge installed with a suitable Python version (3.8 or 3.9). Open your terminal and activate your environment:
conda activate cv
Next, install the required libraries:
conda install -c conda-forge opencv-python face_recognition
With these dependencies installed, we are ready to start coding!
Chapter 2: Extracting Data from Images
For our demonstration, we’ll need three images: two of the same person (let’s use Doctor Strange) and a third image of someone else (Tony Stark).
First, we’ll load and analyze the image using OpenCV. To do this, we will import the library and use the imread function, specifying the path to your script.
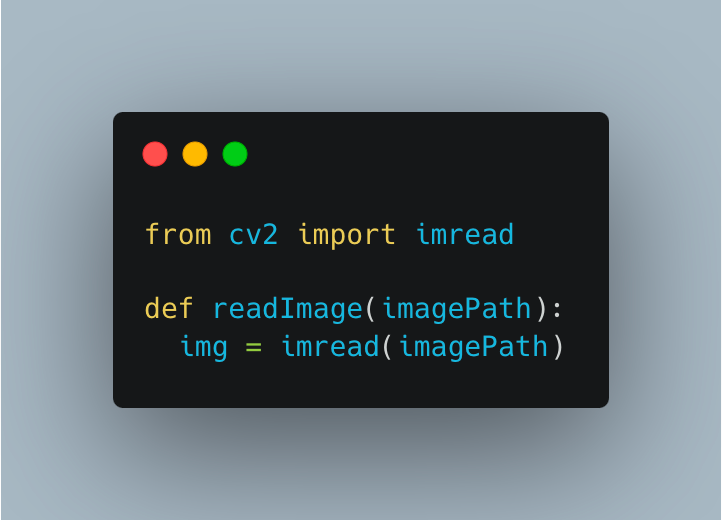
To facilitate face recognition, we need to preprocess the image to simplify the data.
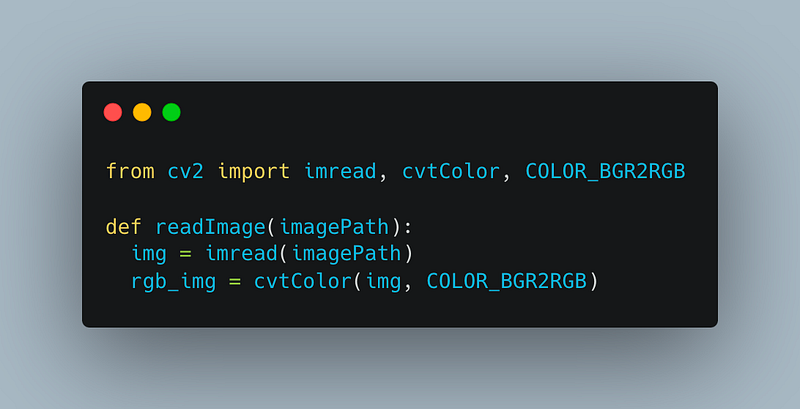
After simplifying the image, we will encode the faces using the face_recognition library to create a data representation of the faces.
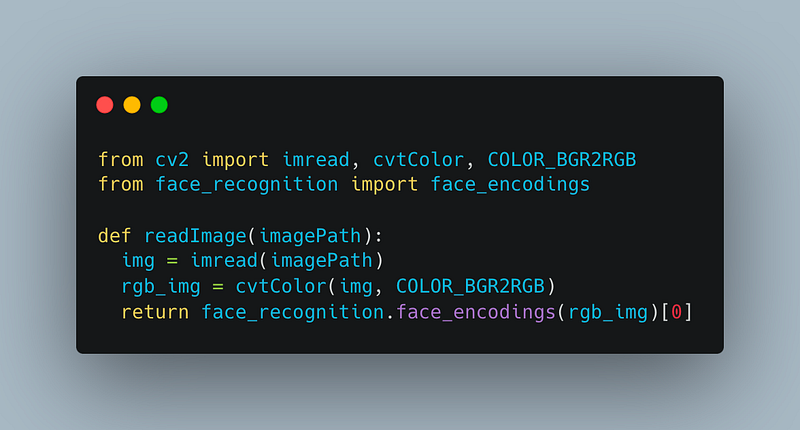
Since we are only processing one face, we will extract the first element from the array of encodings. If you plan to recognize multiple faces, you'll need a different approach.
Comparing the Two Images
Now that we have our readImage function set up, let’s create another function named recognise to compare the two images' data.
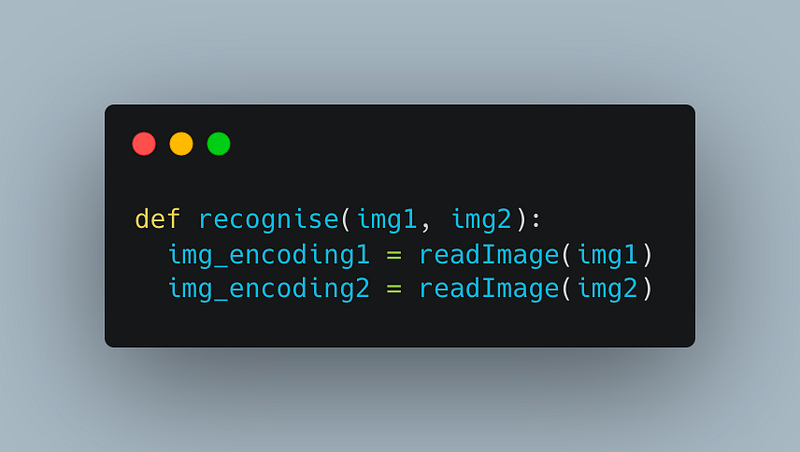
In this function, we will match the encodings of the two images.
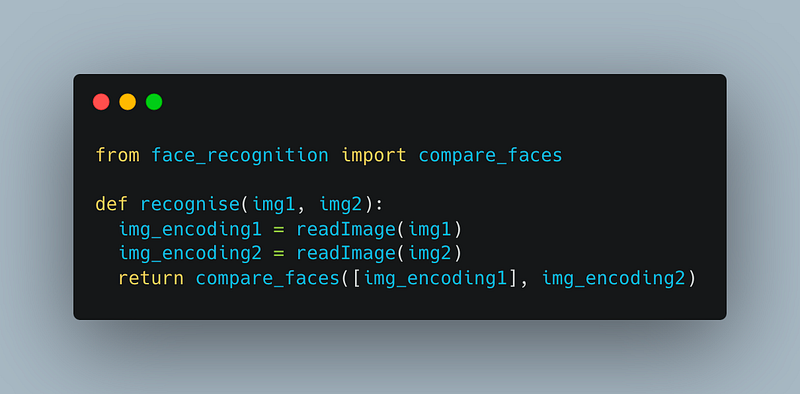
The compare_faces function takes two arguments: an array of encodings and a single encoding. This allows us to compare multiple encodings against one.
Let’s look at the complete code snippet:
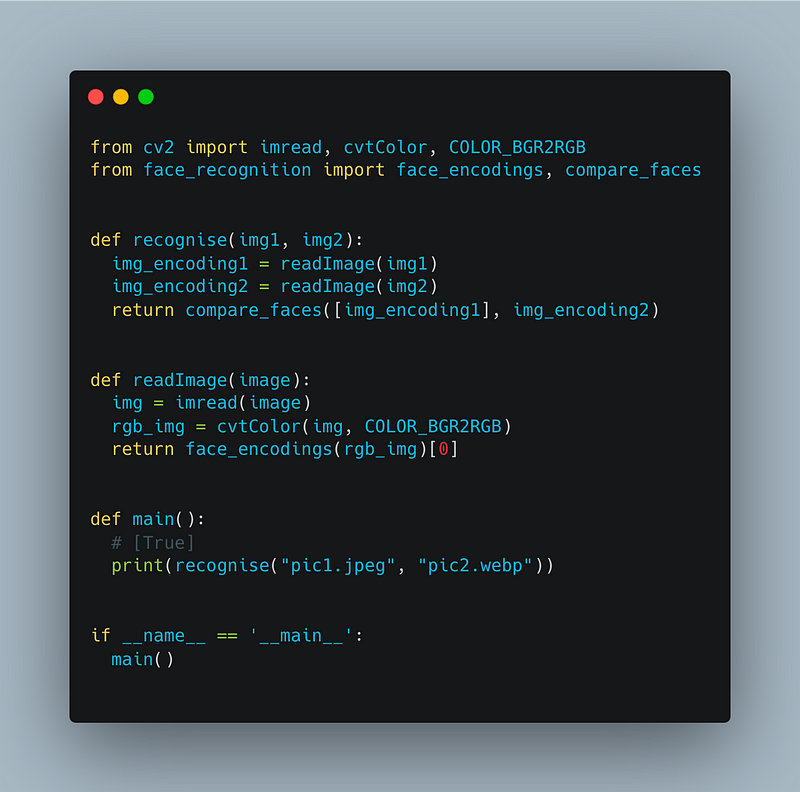
When you run this code, the console will display either [True] or [False], indicating whether the faces match. This method demonstrates how simple it is to implement basic face recognition.
I hope you found this article helpful! If it was useful, please give a clap or follow for more insights!
Chapter 3: Video Resources
To enhance your understanding, check out the following videos:
This video showcases real-time face recognition using OpenCV and Python, providing a visual guide to the concepts discussed.
In this video, you will learn about implementing face recognition with OpenCV and Python, perfect for beginners.