Bridging Data Gaps: An In-Depth Look at the Django-Sequences Library
Written on
Chapter 1: Introduction to Django-Sequences
The django-sequences library provides a powerful solution for producing uninterrupted sequences of integer values.
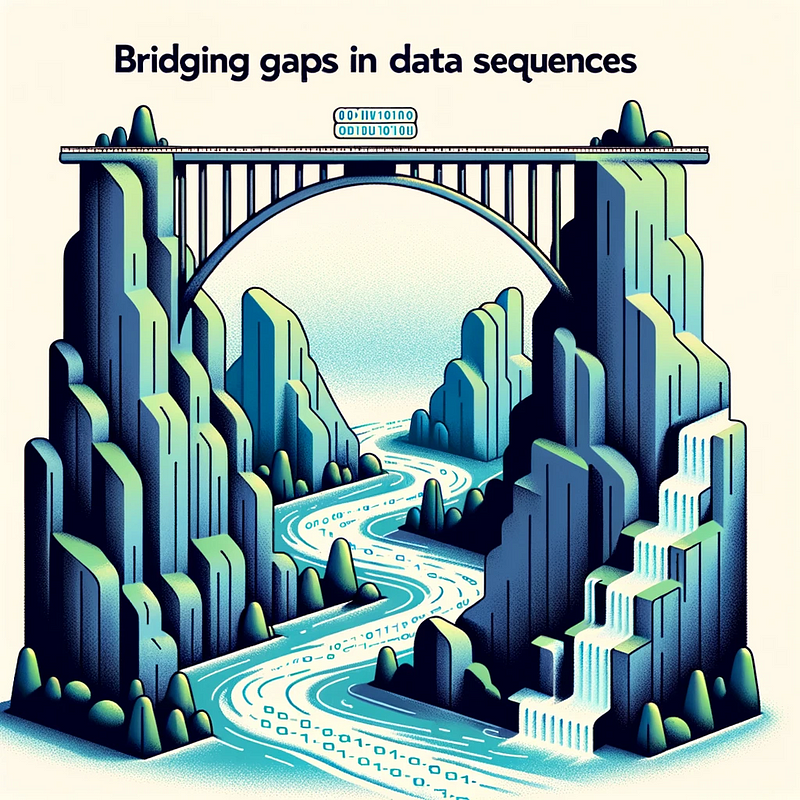
Image by Author
In the realm of web development, maintaining a consistent sequence of identifiers is crucial for numerous applications. Whether it's overseeing invoice numbers in an accounting system or cataloging records in a database, a continuous sequence of integers is essential for preserving data integrity and adhering to various regulatory standards.
Django, a high-level web framework built on Python, accelerates the creation of secure and maintainable sites. However, its default behavior may not adequately support the generation of identifier sequences, particularly when transactions are reverted.
This is where the django-sequences library becomes invaluable, providing a reliable method for generating gapless integer sequences.
For more details, visit the library's GitHub page:
Chapter 2: Understanding Django-Sequences
The django-sequences library is specifically designed to overcome the limitations of Django's default sequence generation. While Django automatically assigns auto-incrementing integers as primary keys for each model, this method does not guarantee a continuous sequence due to potential gaps resulting from transaction rollbacks.
Such gaps can be particularly problematic in fields like accounting, where a consistent sequence of identifiers is vital. The django-sequences library addresses this issue by offering a function called get_next_value, which ensures a gapless sequence of integer values. Additionally, the library ranks among the top 2% of packages on PyPI, underscoring its popularity and effectiveness within the Django ecosystem.
Chapter 3: Utilizing Django-Sequences
Implementing django-sequences is a straightforward process. Start by installing it using the pip command:
pip install django-sequences
Next, add it to the list of applications in your settings.py file:
INSTALLED_APPS = [
...,
"sequences.apps.SequencesConfig",
...
]
Finally, run the migrations:
python manage.py migrate
The primary function, get_next_value, can be employed to maintain a continuous integer sequence. Here’s an example:
from django.db import transaction
from sequences import get_next_value
from invoices.models import Invoice
with transaction.atomic():
Invoice.objects.create(number=get_next_value("invoice_numbers"))
You can specify a sequence name when calling this function. For those who prefer an object-oriented approach, the library offers a Sequence class for managing gapless sequences:
from django.db import transaction
from sequences import Sequence
from invoices.models import Invoice
invoice_numbers = Sequence("invoice_numbers")
with transaction.atomic():
Invoice.objects.create(number=next(invoice_numbers))
The get_next_value function supports multiple independent sequences and allows for the customization of the initial sequence value:
# Generating independent sequences for different use cases
get_next_value("cases") # Output: 1
get_next_value("cases") # Output: 2
get_next_value("invoices") # Output: 1
# Customizing the initial value of a sequence
get_next_value("customers", initial_value=1000) # Output: 1000
Sequences can also be configured to loop, restarting at the initial value after reaching a specified reset_value:
# Looping a sequence
get_next_value("seconds", initial_value=0, reset_value=60) # Sequence restarts at 0 after reaching 59
Additionally, you can create date-specific sequences:
from django.utils import timezone
from sequences import get_next_value
# Per-day sequence
daily_sequence_key = f"books-{timezone.now().date().isoformat()}"
daily_sequence_value = get_next_value(daily_sequence_key)
print(f'Daily sequence value: {daily_sequence_value}')
# Per-year sequence
yearly_sequence_key = f"protocol-{timezone.now().year}"
yearly_sequence_value = get_next_value(yearly_sequence_key)
print(f'Yearly sequence value: {yearly_sequence_value}')
This will generate outputs like books-2023-10-24 or protocol-2023, respectively.
Chapter 4: Conclusion
The django-sequences library stands out as a critical tool for developers using Django, particularly in applications where data integrity and adherence to specific sequencing needs are essential. With its user-friendly API, django-sequences reliably generates gapless integer sequences, effectively filling the gaps inherent in Django's default behavior. Its simplicity and effectiveness in ensuring sequential integrity make django-sequences an essential asset for any developer's toolkit.
Thank you for reading, and I hope to see you online!
Explore additional articles that may interest you:
- Django-log-request-id: Enhancing Logging in Django Applications
- Demystifying Django Slick Reporting: A Comprehensive Guide
- Email Management in Django with Django-Mailbox
This tutorial covers the implementation of sign language detection using Python and Scikit Learn, providing insights into landmark detection and computer vision techniques.