Understanding Python's Call by Sharing Mechanism
Written on
Chapter 1: Introduction to Function Argument Passing in Python
In Python, the terms "call by value" and "call by reference" do not fully capture how function arguments are passed, leading to considerable confusion. Unlike in languages like C or C++, Python utilizes a mechanism known as "call by sharing."
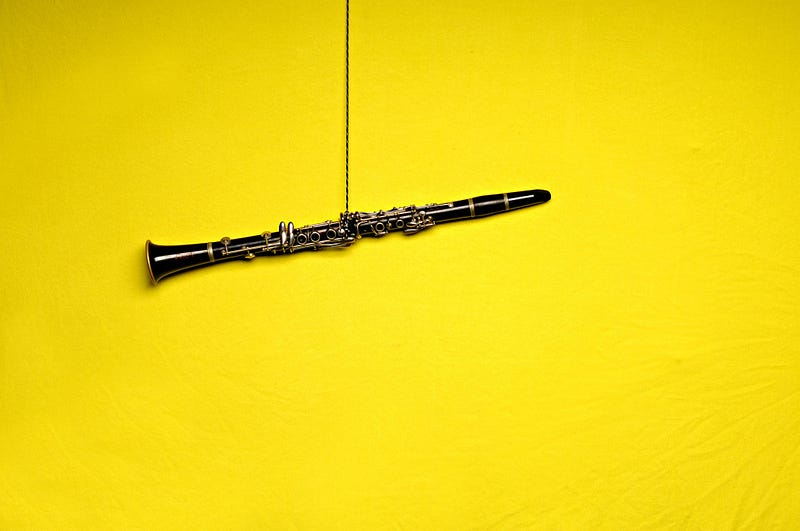
Consider the following example where a function modifies a variable's value:
def test_func(val):
val = val + ' 2024'
print(val)
author = 'Yang Zhou'
test_func(author)
# Output: Yang Zhou 2024
print(author)
# Output: Yang Zhou
In the above code, we observe that while the function alters the variable val, the original variable author remains unchanged. This might lead one to conclude that Python employs call-by-value since it appears that a copy of the object is passed.
Section 1.1: Exploring Object Identity
However, let’s examine this further:
def test_func(val):
print(id(val))
author = 'Yang Zhou'
print(id(author))
# Output: 4336030448
test_func(author)
# Output: 4336030448
Here, the output shows that both val and author share the same memory address. The built-in id() function reveals the memory location of the object. Since val and author point to the same location, it suggests that val is not merely a copy of author.
Subsection 1.1.1: Understanding Python's Object Model
In Python, every variable is essentially a name bound to an object. In this case, val is simply another name for the string "Yang Zhou." When the function attempts to modify val, it creates a new string since strings are immutable in Python. Thus, the original string remains intact.
This leads us to the concept of "call by sharing," which is more accurate than the traditional terms. Here, only certain types of values, referred to as "boxed types" or "references," maintain reference semantics. The address of these objects is passed into the function, but the actual address is a copy. Thus, changes to the parameter do not affect the original variable unless the object is mutable.
Section 1.2: The Implications of Mutability
To illustrate this further, let’s modify our example:
def test_func(val):
val.append('Zhou')
print(val)
print(id(val))
author = ['Yang']
print(id(author))
# Output: 4305358976
test_func(author)
# Output: ['Yang', 'Zhou']
# Output: 4305358976
print(author)
# Output: ['Yang', 'Zhou']
In this case, we converted author from a string to a list. The original list is modified directly, demonstrating that Python behaves like call-by-reference in this situation. However, this does not contradict our previous findings; it rather highlights the nuances of mutability in Python.
The key takeaway is that while both val and author share the same identity, the behavior differs based on whether the data type is mutable or immutable.
Here's a summary table of the mutability of common Python data types:
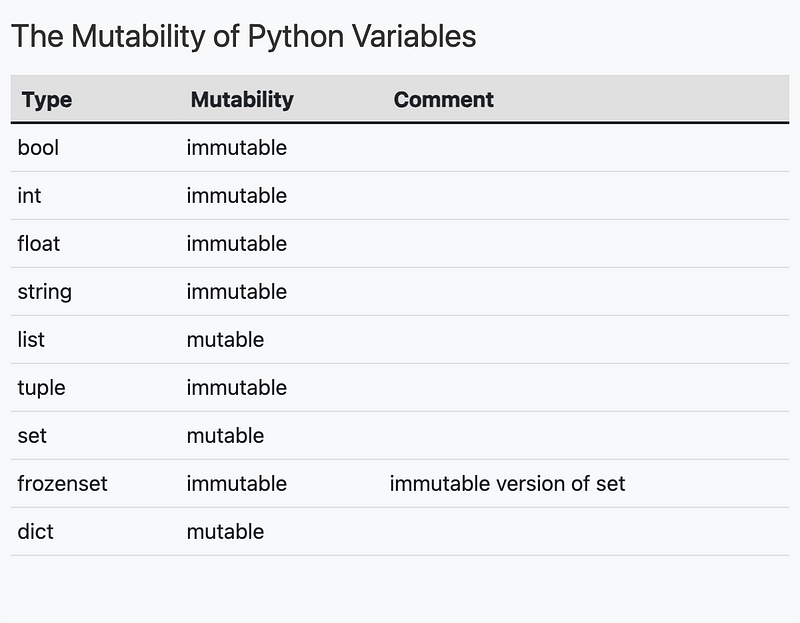
Chapter 2: Conclusion
Understanding how Python handles data types and function parameters is crucial for avoiding subtle bugs in your code. By recognizing the implications of mutability and the call-by-sharing mechanism, you can write more robust and reliable Python applications.
Explore the question: Is Python call by value or call by reference? This video delves into the intricacies of Python's argument passing mechanism.
In this video, we discuss the differences between Pass by Value and Pass by Reference, providing a comprehensive overview within the context of Python.