Understanding the Adapter Design Pattern in Software Development
Written on
Chapter 1: Introduction to the Adapter Pattern
The Adapter pattern is a structural design approach that facilitates the integration of classes and objects into a larger framework. This design pattern enables two disparate objects, which may have incompatible interfaces, to cooperate effectively.
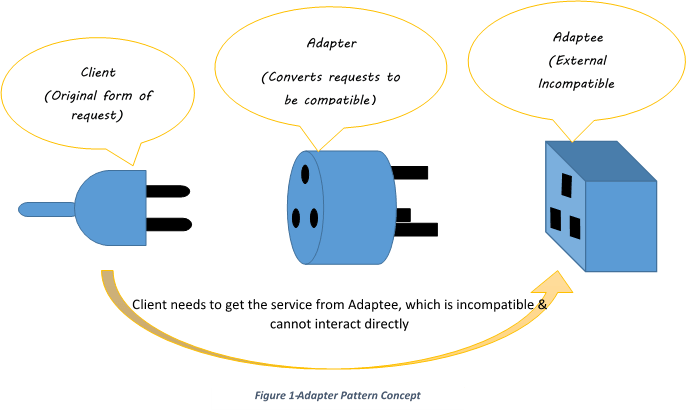
When is the Adapter Pattern Applicable?
- Facilitating Collaboration Between Incompatible Interfaces
The primary use of the Adapter pattern is to enable interaction between objects that have incompatible interfaces. This situation often arises when an object is unsure of which method to invoke due to differing interfaces. The Adapter pattern addresses this challenge by providing an intermediary that conforms to the expected interface, thus simplifying usage. This intermediary, referred to as an adapter, conceals the complexities of the conversion process.
- Integrating Components with Similar Functions
This pattern is also beneficial when two components with distinct interfaces yet similar functionalities need to work together. If their functionalities diverge, the Adapter pattern can modify, add, or remove behaviors, but it should not alter the fundamental purpose of the components. Its role is strictly to transform one interface into another, ensuring seamless communication between disparate systems.
- Decoupling Implementation from Interface
The Adapter pattern is ideal for situations where unrelated interfaces must interact, especially when the needed interface may evolve over time. By utilizing an adapter, we can isolate implementation details from client code, thereby minimizing dependencies and safeguarding the code against changes in the API. The adapter serves as a translator between the current interface and the required one, allowing for modifications without necessitating changes to the client code. This approach enhances extensibility without compromising the existing implementation, aligning with the Open/Closed Principle.
Section 1.1: Practical Example in Swift
To illustrate the Adapter pattern, let’s consider a brief example using Swift. Suppose we are developing an application that displays a list of individuals from a database. The API structure may look like this:
struct Person {
var name: String
var surname: String
var age: Int
var gender: String
}
However, we prefer not to utilize API models directly within our interface layer. Therefore, we need to create a decoupled UI layer:
protocol PersonUI {
var fullName: String { get }
var personAge: Int { get }
}
In this scenario, we will use an instance of PersonUI at the interface layer to act as a converter between the API layer and the UI layer. Thus, we have both an API model and a UI model (the converter) and need to convert the API model to the UI model. By conforming to the protocol, we can easily achieve this:
extension Person: PersonUI {
var fullName: String {
return name + " " + surname}
var personAge: Int {
return age}
}
Summary of the Adapter Pattern
The Adapter pattern serves as a structural design framework that integrates classes and objects into a larger system. It is particularly useful when objects with incompatible interfaces need to collaborate. By transforming one interface into another, the Adapter pattern facilitates communication and reduces dependencies while enhancing client extensibility without modification.
Chapter 2: Video Resources
For further exploration of the Adapter design pattern, consider watching the following videos:
This video provides a comprehensive overview of the Adapter Design Pattern, illustrating its importance and applications in software development.
This episode discusses the Adapter Pattern in detail as part of a broader series on design patterns, providing deeper insights and examples.
Conclusion
Thank you for engaging with this material! I appreciate your support and hope you find the information valuable for your understanding of the Adapter design pattern.