Understanding the Compare the Triplets Problem in Easy Mode
Written on
Introduction to the Triplet Comparison Challenge
In this challenge, Alice and Bob each devised a problem for HackerRank. A reviewer assesses their tasks, providing scores ranging from 1 to 100 across three categories: clarity, originality, and difficulty.
The ratings for Alice’s and Bob’s challenges are represented as triplets: (a = (a[0], a[1], a[2])) for Alice and (b = (b[0], b[1], b[2])) for Bob. The objective is to evaluate their performance by comparing corresponding elements of these triplets.
For each category:
- If (a[i] > b[i]), Alice earns 1 point.
- If (a[i] < b[i]), Bob gains 1 point.
- If (a[i] = b[i]), no points are awarded.
The total points accrued by each participant are referred to as comparison points. The task is to compute these points based on the provided triplets.
Example Scenario
Consider the following example:
- (a = [1, 2, 3])
- (b = [3, 2, 1])
In this case:
- For the first element, Bob scores a point since (a[0] < b[0]).
- No points are awarded for the second element as both scores are equal.
- Finally, Alice secures a point for the third element since (a[2] > b[2]).
The resulting scores are represented as an array: ([1, 1]), where the first value is Alice’s score and the second is Bob’s.
Function Specification
The function compareTriplets should be implemented with the following parameters:
- int a[3]: Alice’s ratings
- int b[3]: Bob’s ratings
It returns an array of two integers: the first element being Alice's score and the second Bob's.
Input Format
The input consists of:
- The first line includes three space-separated integers representing triplet (a).
- The second line contains three space-separated integers for triplet (b).
Constraints: (1 leq a[i], b[i] leq 100)
Sample Input
5 6 7
3 6 10
Sample Output
1 1
Explanation
Alice and Bob both score 1 point each in this scenario.
Analyzing the Problem
This is a straightforward question. The solution should be self-explanatory.
Java Implementation:
public static List<Integer> compareTriplets(List<Integer> a, List<Integer> b) {
List<Integer> score = new ArrayList<Integer>();
int alice = 0;
int bob = 0;
for (int i = 0; i < a.size(); i++){
if(a.get(i) > b.get(i)){
alice++;} else if(a.get(i) < b.get(i)){
bob++;}
}
score.add(alice);
score.add(bob);
return score;
}
Python Implementation:
def compareTriplets(a, b):
first_a = 0
last_b = 0
for i in range(len(a)):
if a[i] > b[i]:
first_a += 1elif a[i] < b[i]:
last_b += 1
return [first_a, last_b]
Kotlin Implementation:
fun compareTriplets(a: Array<Int>, b: Array<Int>): Array<Int> {
var scoreA = 0
var scoreB = 0
for (item in a.indices){
if(a[item] > b[item])
scoreA += 1if(a[item] < b[item])
scoreB += 1}
return arrayOf(scoreA, scoreB)
}
C++ Implementation:
vector<int> compareTriplets(vector<int> a, vector<int> b) {
int alice = 0;
int bob = 0;
for(int i = 0; i < a.size(); i++) {
if(a[i] > b[i]) {
alice++;} else if(a[i] < b[i]) {
bob++;}
}
return vector<int>{alice, bob};
}
Stay tuned for more engaging interview questions as I continue to share insights from my experience as a senior software engineer at MANNG.
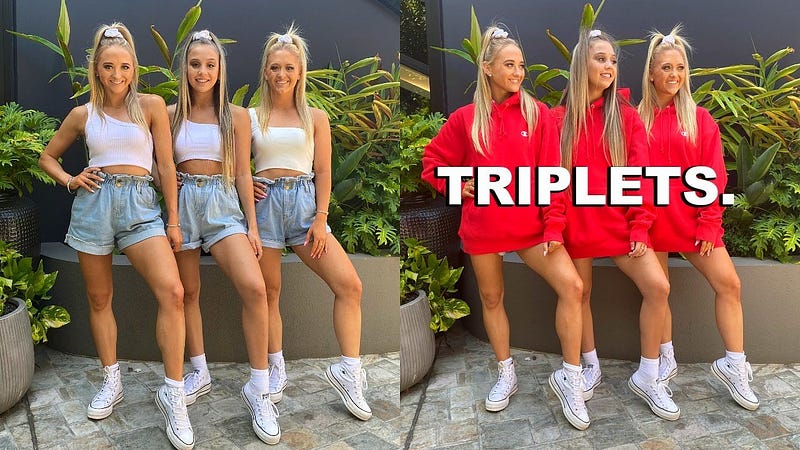
Chapter 2: Video Resources
To deepen your understanding of the Compare the Triplets challenge, check out these helpful video tutorials:
The first video provides a simple approach to the HackerRank solution for the Compare the Triplets challenge.
The second video demonstrates the solution using a ternary operator, offering a concise alternative approach.